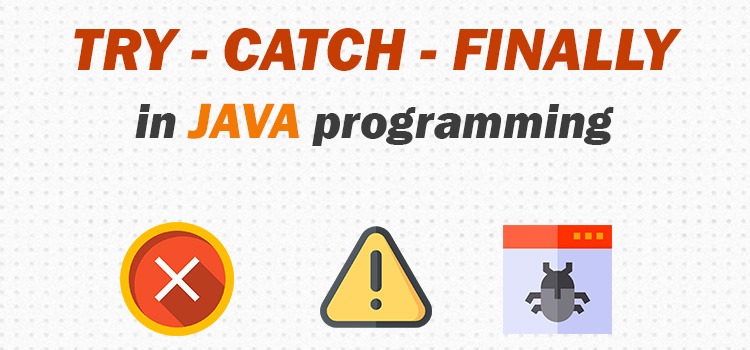
Java is programming language is used to develop web as well as desktop applications. The developers have to compile the code and then execute it. Users get two types of errors in their code which are compile-time, and runtime. Both types of errors can be eliminated by making changes in the code but there are some exceptions that developers can see only at the time of execution.
These exceptions can be eliminated using the try-catch block. If these exceptions will not be handled, the code will compile successfully but will not execute as long as the try-catch block is not used. Let’s know what is try-catch block.
Table of Contents
Try Block
Try block is a block in which these statements are included in which exceptions can occur. There should always be a catch block after the try block which is used to handle the exceptions that may occur due to the statements mentioned on the try block. Another block that can be used after the try block is finally block. The developers can use catch block or finally block or both of them after the try block.
The SYNTAX of the try block is given here.
try
{
//statements
}
If the developers have doubts that a statement may throw an exception, he must use that statement in a try block followed by catch block.
Catch Block
Catch block is a block which is used to handle all the exceptions for the statements mentioned n the try block. A try block can have one or more catch blocks which can handle different exceptions thrown by different statements. There are many types of exceptions that can be handled by catch block.
The SYNTAX of the catch block is given here.
try
{
//Statements
}
catch(exception (type) e(object)
{
//Code for exception handling
}
Example: Try-Catch Block
The developers can use a generic catch block to handle all the exceptions or can use separate catch blocks for each exception. The developers make a note of it that if he is using multiple catch blocks, then the catch block that is generic and can handle all the exceptions should be placed after all the catch blocks are written.
Here is the EXAMPLE of using a try-catch block. In this example, we will handle the Arithmetic Exception.
class TryCatchExample
{
try
{
int num1, num2, num3;
num1 = 50
num2 = 0
num3 = num1/num2
System.out.println(num3);
}
catch(Arithmetic Exception e)
{
System.out.println(“A number cannot be divided by zero”);
}
catch(Exception e)
{
System.out.println(“The code has exception”);
}
}
In this example, there are statements in the try block in which a number is being divided by zero. There are two catch blocks in the code.
In the first catch block, the arithmetic exception has been handled and in the second block all the exceptions are handled. The developers must notice that the generic catch block has been included in the last.
Multiple Catch Blocks in Java
As mentioned earlier, users can use multiple catch blocks for one try block. In spite of a generic exception handling catch block, developers need separate catch blocks for each exception because the generic one will display same message for all the exceptions but separate catch block will display messages for different types of exceptions. The catch blocks are not executed if no exception is thrown from the try block.
Example of Multiple Catch Blocks
Here is another example in which we will use separate catch blocks to handle Arithmetic Exception ArryIndexOutOfBoundsException and generic catch block.
class ExceptionExample
{
public static void main(String args[]){
try
{
int a[]=new int[6];
a[4]=28/0;
System.out.println("First print statement in try block");
}
catch(ArithmeticException e)
{
System.out.println("Warning: ArithmeticException");
}
catch(ArrayIndexOutOfBoundsException e)
{
System.out.println("Warning: ArrayIndexOutOfBoundsException");
}
catch(Exception e)
{
System.out.println("Warning: Some Other exception");
}
System.out.println("Out of try-catch block...");
}
}
Finally Block
The
When a developer is working on file handling, he enters and exits the files through the code. After exiting the file, there is no need for exception handling. In such a case
Here is an EXAMPLE of using finally block.
class FinallyExample
{
public static void main(String args[])
{
try
{
Int num1, num2, num3;
num1 = 40;
num2 = 0;
num3 = num1/num2;
System.out.println(num3);
}
finally
{
System.out.prontln(“exception occurred);
}
}
}
Java Exception Class Hierarchy
There are many exception classes which users can use to handle if an exception occurs. The exception classes can be found in java.lang package. Here is the list of exception classes.
- ArithmeticException
- ArrayIndexOutOfBoundsException
- ArrayStoreException
- ClassCastException
- EnumConstantNotPresentException
- IllegalArgumentException
- IllegalMonitorStateException
- IllegalStateException
- IllegalThreadStateException
- IndexOutOfBoundsException
- NegativeArraySizeException
- NullPointerException
- NumberFormatException
- SecurityException
- StringIndexOutOfBounds
- TypeNotPresentException
- UnsupportedOperationException
- ClassNotFoundException
- CloneNotSupportedException
- IllegalAccessException
- InstantiationException
- InterruptedException
- NoSuchFieldException
- NoSuchMethodException
The developers can also create their own exception classes if needed. In order to create the user-defined exception class, the developers have to define a subclass that extends Exception class. There are no methods in the Exception class but it can inherit the methods from the Throwable class.
Wrap Up
In this article we have discussed the methods of using try, catch, and finally blocks in order to handle the exceptions. The developers can use the try-catch block to handle any type of exception whether it is related to arithmetic calculation, arrays, file handling, and many others.
The exception handling is necessary if the code has to be executed. If the exceptions are not handled, then either the code will show the runtime error during execution or the code will show abrupt output. So developers must know the value of the try-catch block and use them to handle exceptions.